Tailwind CSS Countdown Timer
A countdown timer in Tailwind CSS is a UI component used to display a dynamically decreasing time interval. It can be used to create a sense of urgency or to indicate the time remaining until a specific event or deadline.
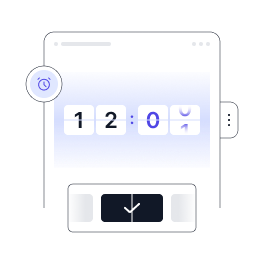
Countdown timer display area, where the remaining time is shown. This display area may include digits representing hours, minutes, seconds, and milliseconds in the countdown.
You can customize the design and its appearance to match the design and branding of your application. To change the time of the countdown, you can customize the date and time according to your requirements.
The countdown timer requires a start time, indicating when the countdown should begin, and, optionally, an end time, indicating when the countdown should end.
Add tailwind coutdown into your website with pagedone customizable
classes. You have to follow few steps to add classes in your 'javascript file'
file where you define your
project's customized scripts.
- Add below script in 'javascript' file in your project.
- Use customized Countdown Timer classes shown below.
<script>
// count-down timer
let dest = new Date("apr 25, 2024 10:00:00").getTime();
let x = setInterval(function () {
let now = new Date().getTime();
let diff = dest - now;
// Check if the countdown has reached zero or negative
if (diff <= 0) {
clearInterval(x); // Stop the countdown
return; // Exit the function
}
let days = Math.floor(diff / (1000 * 60 * 60 * 24));
let hours = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
let minutes = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60));
let seconds = Math.floor((diff % (1000 * 60)) / 1000);
if (days < 10) {
days = `0${days}`;
}
if (hours < 10) {
hours = `0${hours}`;
}
if (minutes < 10) {
minutes = `0${minutes}`;
}
if (seconds < 10) {
seconds = `0${seconds}`;
}
// Get elements by class name
let countdownElements = document.getElementsByClassName("countdown-element");
// Loop through the elements and update their content
for (let i = 0; i < countdownElements.length; i++) {
let className = countdownElements[i].classList[1]; // Get the second class name
switch (className) {
case "days":
countdownElements[i].innerHTML = days;
break;
case "hours":
countdownElements[i].innerHTML = hours;
break;
case "minutes":
countdownElements[i].innerHTML = minutes;
break;
case "seconds":
countdownElements[i].innerHTML = seconds;
break;
default:
break;
}
}
}, 1000);
</script>
1000+ Tailwind Blocks
Access over 1,000 ready-made Tailwind blocks with modern designs to accelerate your design process.
Explore More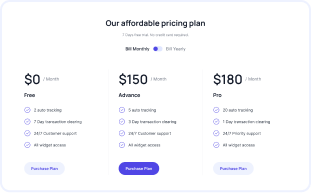
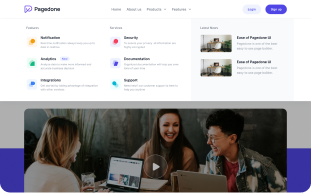
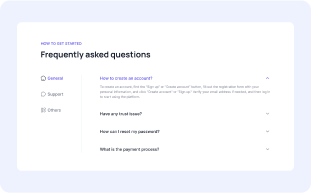
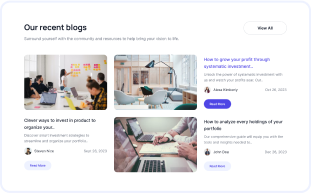
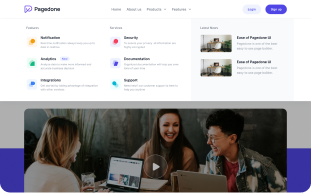
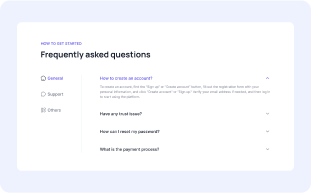
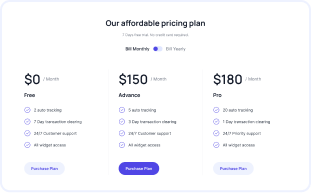
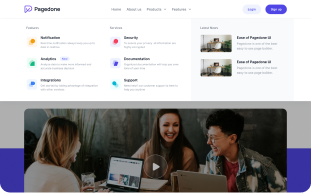
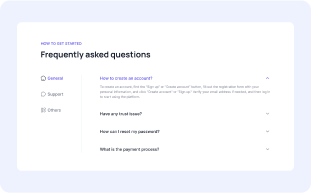
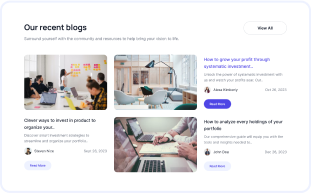
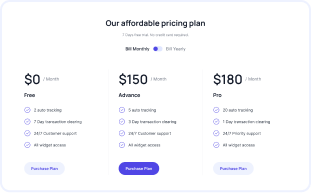
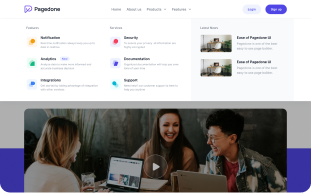
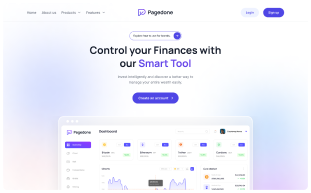
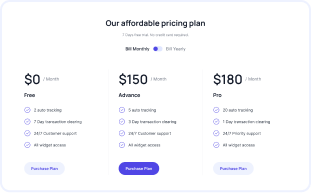
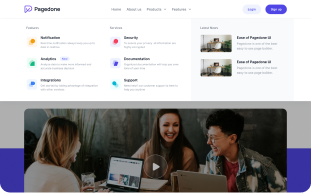
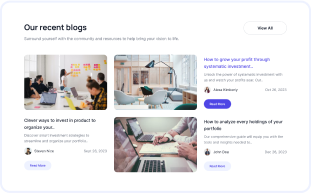
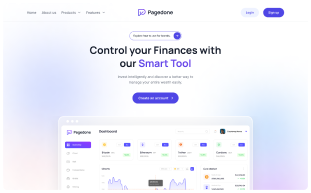
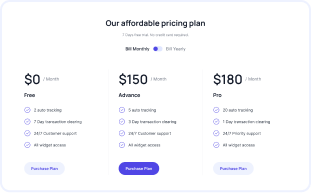
Custom Tailwind Countdown Timer
Below are list of customized Countdown Timer with their class names.
Default countdown timer
Use this default tailwind countdown, which shows the remaining time for a specific event in a box-style layout. Here, you can customize the date and time according to your needs.
days
hours
minutes
seconds
<div class="flex items-center justify-center w-full gap-6 count-down-main">
<div class="timer">
<div
class="pr-1.5 pl-2 relative bg-indigo-50 w-max before:contents-[''] before:absolute before:h-full before:w-0.5 before:top-0 before:left-1/2 before:-translate-x-1/2 before:bg-white before:z-10 ">
<h3
class="countdown-element days font-manrope font-semibold text-2xl text-indigo-600 tracking-[15.36px] max-w-[44px] text-center relative z-20"
>
</h3>
</div>
<p class="text-sm font-normal text-gray-900 mt-1 text-center w-full">days</p>
</div>
<div class="timer">
<div
class="pr-1.5 pl-2 relative bg-indigo-50 w-max before:contents-[''] before:absolute before:h-full before:w-0.5 before:top-0 before:left-1/2 before:-translate-x-1/2 before:bg-white before:z-10 ">
<h3
class="countdown-element hours font-manrope font-semibold text-2xl text-indigo-600 tracking-[15.36px] max-w-[44px] text-center relative z-20"
>
</h3>
</div>
<p class="text-sm font-normal text-gray-900 mt-1 text-center w-full">hours</p>
</div>
<div class="timer">
<div
class="pr-1.5 pl-2 relative bg-indigo-50 w-max before:contents-[''] before:absolute before:h-full before:w-0.5 before:top-0 before:left-1/2 before:-translate-x-1/2 before:bg-white before:z-10 ">
<h3
class="countdown-element minutes font-manrope font-semibold text-2xl text-indigo-600 tracking-[15.36px] max-w-[44px] text-center relative z-20"
>
</h3>
</div>
<p class="text-sm font-normal text-gray-900 mt-1 text-center w-full">minutes</p>
</div>
<div class="timer">
<div
class="pr-1.5 pl-2 relative bg-indigo-50 w-max before:contents-[''] before:absolute before:h-full before:w-0.5 before:top-0 before:left-1/2 before:-translate-x-1/2 before:bg-white before:z-10 ">
<h3
class="countdown-element seconds font-manrope font-semibold text-2xl text-indigo-600 tracking-[15.36px] max-w-[44px] text-center relative z-20"
>
</h3>
</div>
<p class="text-sm font-normal text-gray-900 mt-1 text-center w-full">seconds</p>
</div>
</div>
Countdown with seprator
The following example of a countdown in tailwind shows days, hours, minutes, and seconds separated from each other by a specific sign.
days
:
hours
:
minutes
:
seconds
<div class="flex items-start justify-center w-full gap-4 count-down-main">
<div class="timer w-16">
<div
class="">
<h3>
class="countdown-element days font-manrope font-semibold text-2xl text-indigo-600 text-center"
>
</h3>
</div>
<p> class="text-sm font-normal text-gray-900 mt-1 text-center w-full">days</p>
</div>
<h3> class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class="">
<h3>
class="countdown-element hours font-manrope font-semibold text-2xl text-indigo-600 text-center"
>
</h3>
</div>
<p> class="text-sm font-normal text-gray-900 mt-1 text-center w-full">hours</p>
</div>
<h3> class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class="">
<h3>
class="countdown-element minutes font-manrope font-semibold text-2xl text-indigo-600 text-center"
>
</h3>
</div>
<p> class="text-sm font-normal text-gray-900 mt-1 text-center w-full">minutes</p>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class="">
<h3>
class="countdown-element seconds font-manrope font-semibold text-2xl text-indigo-600 text-center"
>
</h3>
</div>
<p> class="text-sm font-normal text-gray-900 mt-1 text-center w-full">seconds</p>
</div>
</div>
Tailwind countdown timer with animation
The below example shows days, hours, minutes, and seconds in an animated way. You can change the background color according to your needs.
days
hours
minutes
seconds
<div class="flex items-start justify-center w-full gap-4 count-down-main">
<div class="timer w-16">
<div
class=" bg-indigo-600 py-4 px-2 rounded-lg overflow-hidden">
<h3
class="countdown-element days font-Cormorant font-semibold text-2xl text-white text-center">
</h3>
</div>
<p class="text-lg font-Cormorant font-medium text-gray-900 mt-1 text-center w-full">days</p>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class=" bg-indigo-600 py-4 px-2 rounded-lg overflow-hidden">
<h3
class="countdown-element hours font-Cormorant font-semibold text-2xl text-white text-center">
</h3>
</div>
<p class="text-lg font-Cormorant font-normal text-gray-900 mt-1 text-center w-full">hours</p>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class=" bg-indigo-600 py-4 px-2 rounded-lg overflow-hidden">
<h3
class="countdown-element minutes font-Cormorant font-semibold text-2xl text-white text-center">
</h3>
</div>
<p class="text-lg font-Cormorant font-normal text-gray-900 mt-1 text-center w-full">minutes</p>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer w-16">
<div
class=" bg-indigo-600 py-4 px-2 rounded-lg overflow-hidden ">
<h3
class="countdown-element seconds font-Cormorant font-semibold text-2xl text-white text-center animate-countinsecond">
</h3>
</div>
<p class="text-lg font-Cormorant font-normal text-gray-900 mt-1 text-center w-full">seconds</p>
</div>
</div>
1000+ Tailwind Blocks
Access over 1,000 ready-made Tailwind blocks with modern designs to accelerate your design process.
Explore More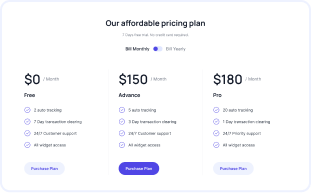
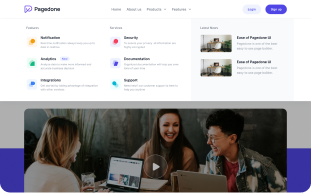
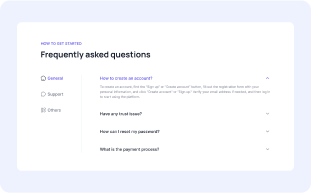
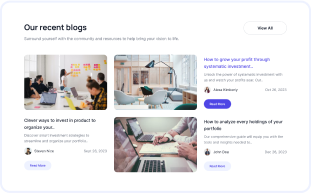
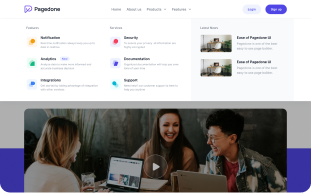
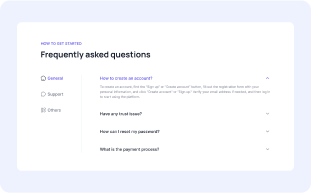
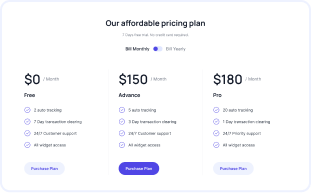
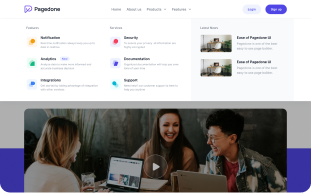
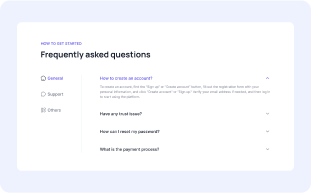
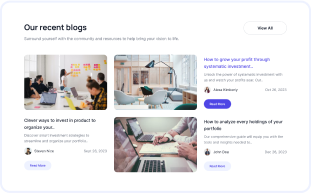
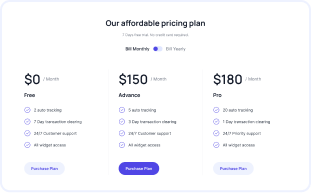
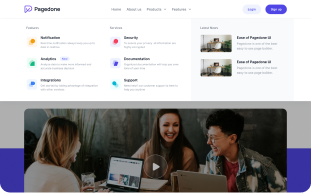
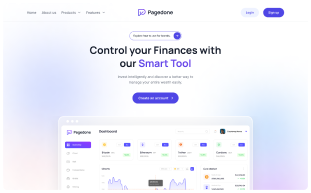
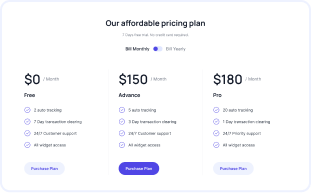
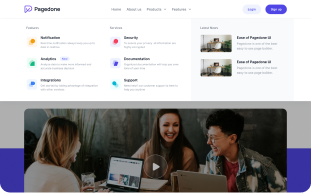
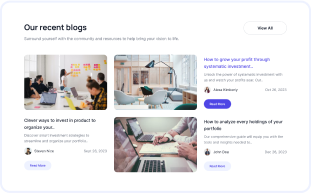
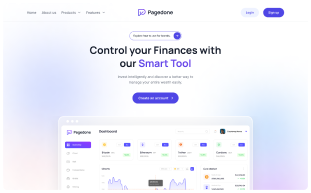
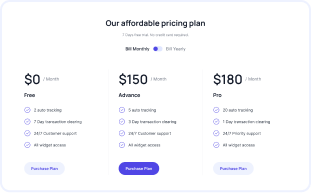
Countdown timer with a gradient background
This customizable countdown timer in Tailwind shows days, hours, minutes, and seconds inside a gradient background. You can also change gradient colors.
days
:
Hour
:
Minutes
:
Seconds
<div class="flex items-center justify-center w-full gap-1.5 count-down-main">
<div class="timer">
<div
class="rounded-xl bg-gradient-to-b from-indigo-600 to-purple-600 py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 aspect-square px-3">
<h3>
class="countdown-element days font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p> class="text-lg font-manrope font-normal text-white mt-1 text-center w-full">days</p>
</div>
</div>
<h3> class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl bg-gradient-to-b from-indigo-600 to-purple-600 py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 aspect-square px-3">
<h3>
class="countdown-element hours font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p> class="text-lg font-manrope font-normal text-white mt-1 text-center w-full">Hour</p>
</div>
</div>
<h3> class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl bg-gradient-to-b from-indigo-600 to-purple-600 py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 aspect-square px-3">
<h3>
class="countdown-element minutes font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p> class="text-lg font-manrope font-normal text-white mt-1 text-center w-full">Minutes</p>
</div>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl bg-gradient-to-b from-indigo-600 to-purple-600 py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 aspect-square px-3">
<h3>
class="countdown-element seconds font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p> class="text-lg font-manrope font-normal text-white mt-1 text-center w-full">Seconds</p>
</div>
</div>
</div>
Countdown with a blur effect
Use this tailwind CSS countdown to add a blur effect to the background inside the countdown timer. You can use it by adding the following class to your code:.
days
Hour
Minutes
Seconds
<div class="w-full h-96 rounded-2xl flex gap-9 flex-col items-center justify-center bg-cover bg-center" style="background-image: url(https://pagedone.io/asset/uploads/1710565658.jpg);">
<div class="flex items-start justify-center w-full gap-1.5 count-down-main">
<div class="timer">
<div
class="rounded-xl bg-black/25 backdrop-blur-sm py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 px-3">
<h3
class="countdown-element days font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p class="text-lg uppercase font-normal text-white mt-1 text-center w-full">days</p>
</div>
</div>
<div class="timer">
<div
class="rounded-xl bg-black/25 backdrop-blur-sm py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 px-3">
<h3
class="countdown-element hours font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p class="text-lg uppercase font-normal text-white mt-1 text-center w-full">Hour</p>
</div>
</div>
<div class="timer">
<div
class="rounded-xl bg-black/25 backdrop-blur-sm py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 px-3">
<h3
class="countdown-element minutes font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p class="text-lg fo uppercasent-normal text-white mt-1 text-center w-full">Minutes</p>
</div>
</div>
<div class="timer">
<div
class="rounded-xl bg-black/25 backdrop-blur-sm py-3 min-w-[96px] flex items-center justify-center flex-col gap-1 px-3">
<h3
class="countdown-element seconds font-manrope font-semibold text-2xl text-white text-center">
</h3>
<p class="text-lg fo uppercasent-normal text-white mt-1 text-center w-full">Seconds</p>
</div>
</div>
</div>
</div>
Countdown with background
Use this simple example of a countdown with a background color. You can also change the background according to your brand designs.
days
:
Hour
:
Minutes
:
Seconds
<div class="flex rounded-xl items-center justify-center w-max mx-auto p-3 gap-1.5 count-down-main bg-indigo-50">
<div class="timer">
<div
class="rounded-xl py-2 flex items-center justify-center flex-col aspect-square px-1 w-20">
<h3>
class="countdown-element days font-manrope font-semibold text-xl text-indigo-600 text-center">
</h3>
<p> class="text-lg font-Cormorant font-normal text-indigo-600 text-center w-full">days</p>
</div>
</div>
<h3> class="font-manrope font-semibold text-2xl text-indigo-600">:</h3>
<div class="timer">
<div
class="rounded-xl py-2 flex items-center justify-center flex-col aspect-square px-1 w-20">
<h3>
class="countdown-element hours font-manrope font-semibold text-xl text-indigo-600 text-center">
</h3>
<p> class="text-lg font-Cormorant font-normal text-indigo-600 text-center w-full">Hour</p>
</div>
</div>
<h3> class="font-manrope font-semibold text-2xl text-indigo-600">:</h3>
<div class="timer">
<div
class="rounded-xl py-2 flex items-center justify-center flex-col aspect-square px-1 w-20">
<h3>
class="countdown-element minutes font-manrope font-semibold text-xl text-indigo-600 text-center">
</h3>
<p> class="text-lg font-Cormorant font-normal text-indigo-600 text-center w-full">Minutes</p>
</div>
</div>
<h3> class="font-manrope font-semibold text-2xl text-indigo-600">:</h3>
<div class="timer">
<div
class="rounded-xl py-2 flex items-center justify-center flex-col aspect-square px-1 w-20">
<h3>
class="countdown-element seconds font-manrope font-semibold text-xl text-indigo-600 text-center">
</h3>
<p> class="text-lg font-Cormorant font-normal text-indigo-600 text-center w-full">Seconds</p>
</div>
</div>
</div>
Countdown timer with borders
Use this example to add a border to timing boxes and give them a simple look.
days
:
Hour
:
Minutes
:
Seconds
<div class="flex items-center justify-center w-full gap-1.5 count-down-main">
<div class="timer">
<div
class="rounded-xl border border-indigo-600 py-1.5 min-w-[80px] flex items-center justify-center flex-col gap-0 aspect-square px-1.5">
<h3
class="countdown-element days font-manrope font-semibold text-2xl text-indigo-600 text-center">
</h3>
<p class="text-sm font-inter capitalize font-normal text-indigo-600 text-center w-full">days</p>
</div>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl border border-indigo-600 py-1.5 min-w-[80px] flex items-center justify-center flex-col gap-0 aspect-square px-1.5">
<h3
class="countdown-element hours font-manrope font-semibold text-2xl text-indigo-600 text-center">
</h3>
<p class="text-sm font-inter capitalize font-normal text-indigo-600 text-center w-full">Hour</p>
</div>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl border border-indigo-600 py-1.5 min-w-[80px] flex items-center justify-center flex-col gap-0 aspect-square px-1.5">
<h3
class="countdown-element minutes font-manrope font-semibold text-2xl text-indigo-600 text-center">
</h3>
<p class="text-sm font-inter capitalize font-normal text-indigo-600 text-center w-full">Minutes</p>
</div>
</div>
<h3 class="font-manrope font-semibold text-2xl text-gray-900">:</h3>
<div class="timer">
<div
class="rounded-xl border border-indigo-600 py-1.5 min-w-[80px] flex items-center justify-center flex-col gap-0 aspect-square px-1.5">
<h3
class="countdown-element seconds sec font-manrope font-semibold text-2xl text-indigo-600 text-center">
</h3>
<p class="text-sm font-inter capitalize font-normal text-indigo-600 text-center w-full">Seconds</p>
</div>
</div>
</div>
1000+ Tailwind Blocks
Access over 1,000 ready-made Tailwind blocks with modern designs to accelerate your design process.
Explore More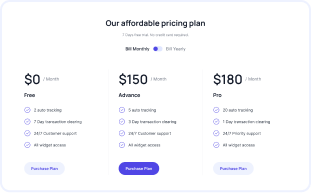
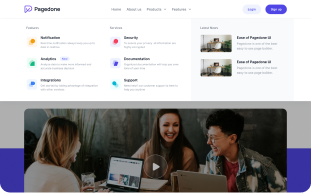
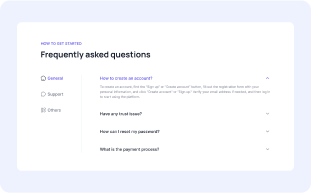
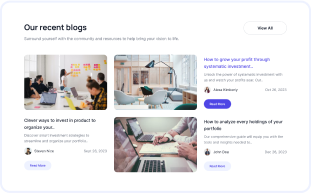
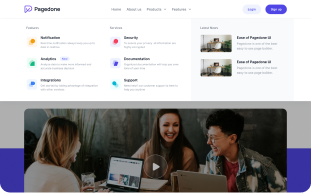
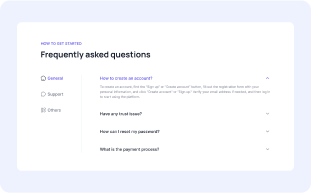
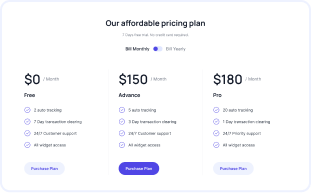
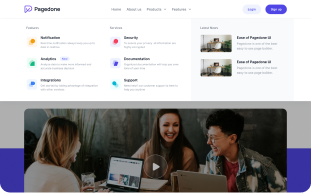
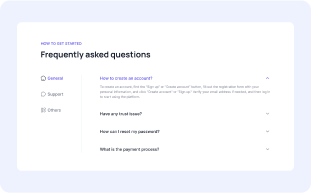
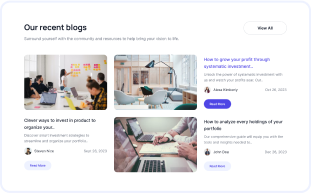
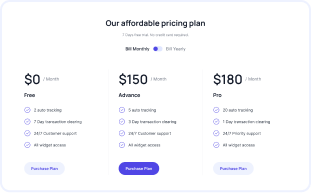
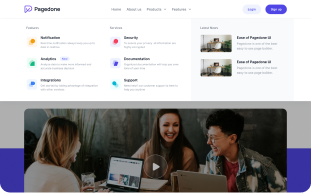
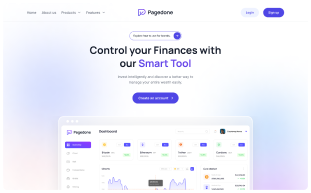
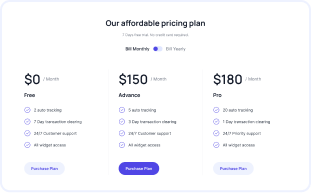
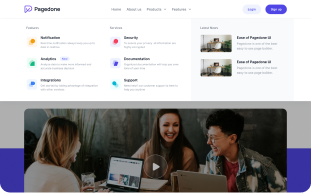
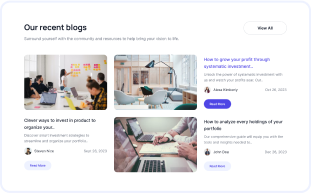
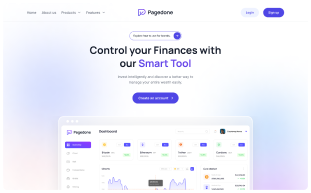
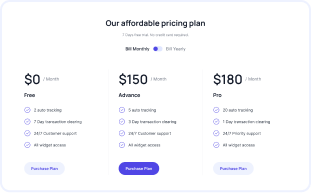